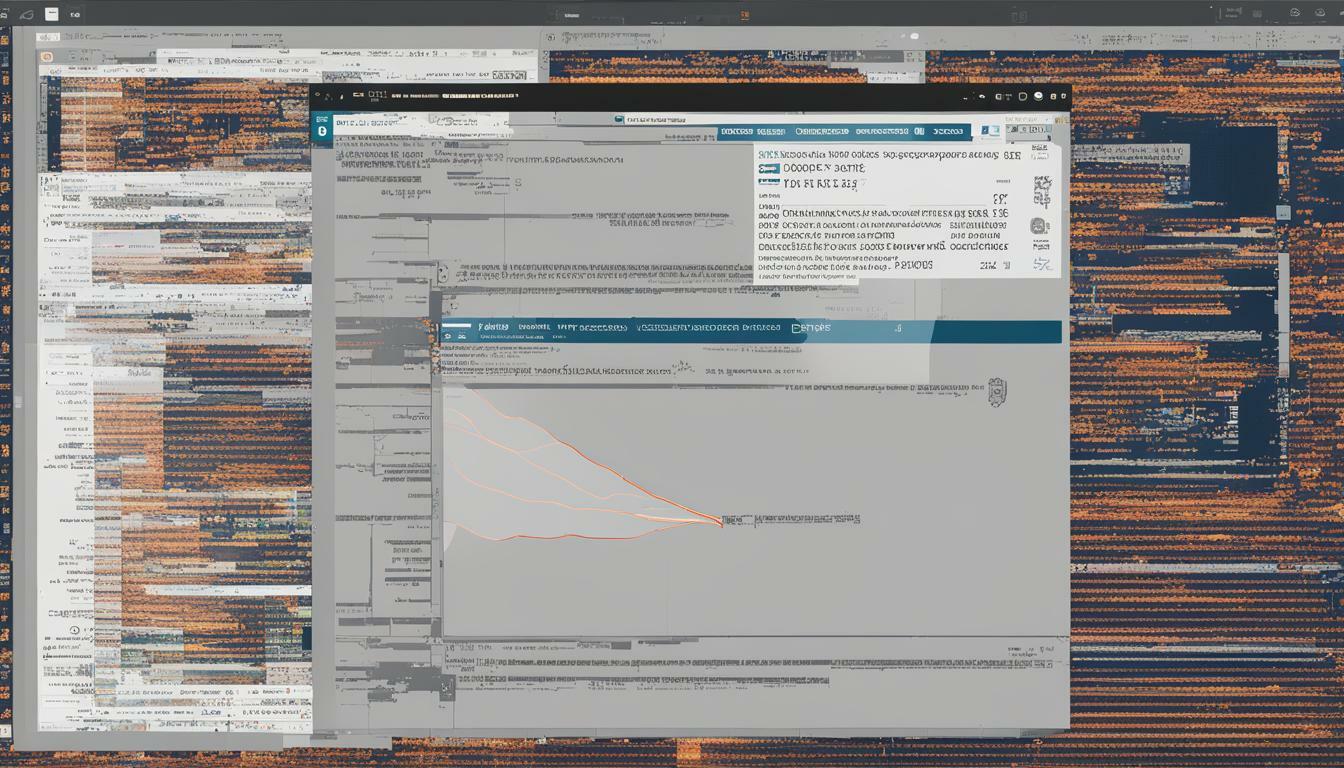
As a developer, you understand the importance of writing clean, efficient code. Code optimization is the process of fine-tuning your code to make it run faster, consume less memory, and perform better overall. In this article, we will explore various tweaks and tricks that can help you optimize your code and achieve better performance.
Whether you’re a seasoned developer or just starting, optimizing your code is essential to improve efficiency and productivity. By implementing code optimization techniques, you can reduce execution time, minimize resource usage, and enhance scalability. Let’s dive in and discover how you can optimize your code for better performance.
Key Takeaways
- Code optimization is crucial for achieving better performance and should be incorporated into your development workflow.
- Efficient code can improve execution speed, reduce memory usage, and enhance scalability.
- Identifying performance bottlenecks and implementing code optimization techniques can significantly improve code efficiency.
Understanding Code Optimization and its Benefits
Code optimization is the process of improving the performance of your code by making tweaks and implementing techniques that enhance its efficiency. When done properly, code optimization can significantly improve execution speed, reduce memory usage, and enhance scalability of your code.
Better performance is the ultimate goal of code optimization. By optimizing your code, you can achieve faster load times and response times, reduced utilization of system resources, and improved user experience. Additionally, when your code is optimized, it can run more efficiently on various devices and platforms, ensuring a better experience for your users.
Improving code efficiency is another key benefit of code optimization. When your code runs more efficiently, it can perform tasks with less redundancy, resulting in reduced overhead and increased productivity. This can translate into significant cost savings, especially when dealing with large-scale projects and applications.
Code optimization achieves all these benefits by making tweaks and modifications that eliminate performance bottlenecks. By identifying areas of your code that can be improved and implementing optimization techniques, you can maximize the efficiency of your code, reduce memory usage, and eliminate unnecessary I/O operations.
In short, code optimization is an essential aspect of software development that can help you achieve better performance, enhance code efficiency, and reduce overhead costs. By staying up-to-date with the latest optimization techniques and tools, you can ensure that your code is optimized for peak performance and long-term success.
Identifying Performance Bottlenecks and Areas for Improvement
Improving code efficiency requires a thorough understanding of the performance bottlenecks that exist in your code. Identifying these bottlenecks is the first step in implementing code optimization techniques that will maximize efficiency. Fortunately, there are several tools and techniques that can help you diagnose and correct performance issues.
Profiling
Profiling is a technique that helps identify performance bottlenecks by analyzing the execution time of different parts of your code. By identifying which parts of your code are taking the longest to execute, you can focus your optimization efforts on those areas. There are several profiling tools available, including PyCharm, Visual Studio, and Xdebug.
Benchmarking
Benchmarking involves testing the performance of your code under different conditions to identify areas that require optimization. This technique is particularly useful for identifying performance bottlenecks in algorithms and data structures. There are several benchmarking tools available, including Apache JMeter, Siege, and Gatling.
Code Review
Code review is an effective technique for identifying performance issues that may be missed by automated tools. By having other developers review your code, you can identify areas that can be optimized for better performance. Additionally, code review can help identify potential bugs and security vulnerabilities in your code.
Debugging
Debugging is a process of identifying and resolving errors in your code. While debugging is not directly related to performance optimization, it is an important step in identifying issues in your code that may be impacting performance.
In conclusion, identifying performance bottlenecks in your code is crucial for achieving maximum efficiency. By using techniques like profiling, benchmarking, code review, and debugging, you can diagnose and resolve performance issues that may be impacting your application’s performance. In the next section, we will explore practical code optimization techniques that can be used to improve performance.
Implementing Code Optimization Techniques
Now that you understand the importance of code optimization for achieving better performance, it’s time to dive into practical tips and techniques for improving your code’s efficiency.
Algorithm Optimizations
One of the most effective techniques for optimizing code performance is to optimize your algorithms. This involves analyzing the complexity of algorithms and finding ways to reduce the number of operations required for a given task. For example, using binary search instead of linear search for operations on large arrays can significantly improve execution speed.
Data Structure Optimizations
Data structure optimizations involve choosing the right data structure for a given task. For example, using a hash table instead of an array for associative data access can improve performance. Additionally, using efficient data structures like balanced trees and heaps can improve the speed of operations like searching and sorting.
Caching Strategies
Caching is a technique used to store frequently accessed data in a faster location for easier retrieval. Caching can help improve performance by reducing the number of I/O operations required. Consider using caching for frequently accessed data that doesn’t change often, such as configuration files or database records.
Minimizing I/O Operations
Input/output (I/O) operations can be resource-intensive and can significantly impact the performance of your code, especially when dealing with large files or network requests. To optimize code performance, minimize the number of I/O operations, use buffered I/O, and reduce the amount of data that needs to be read or written.
Reducing Code Redundancy
Code redundancy can also impact code performance. Eliminate duplicate code by using functions or classes to reduce the amount of code that needs to be executed. Additionally, avoid using unnecessary variables, loops, and conditional statements that can slow down your code.
By applying these code optimization techniques, you can significantly improve the efficiency and performance of your code, resulting in faster execution speeds, reduced memory usage, and enhanced scalability.
Testing and Measuring Performance Improvements
Once you have implemented various code optimization techniques, it is crucial to test and measure the performance improvements achieved. This allows you to determine the effectiveness of the optimizations and make further improvements if necessary.
The Importance of Performance Enhancements
Performance enhancements are critical for ensuring that your software runs efficiently, providing the best possible user experience. By optimizing your code, you can improve execution speed, reduce memory usage, and enhance scalability.
Tools and Methodologies for Performance Measurement
There are several tools and methodologies available for measuring the performance of your code. Load testing, stress testing, and profiling are some examples that can help you analyze the effect of optimizations on your code performance. These tools and techniques can provide you with valuable insights into the impact of your optimizations and help you identify areas for further improvement.
Interpreting Performance Metrics
Interpreting performance metrics can be challenging, but it is fundamental for evaluating the effectiveness of your code optimizations. You can use metrics such as response time, throughput, and error rate to quantify the performance of your code. Analyzing the metrics and comparing them with the baseline can help you determine the impact of your optimizations and measure the improvements achieved.
In summary, testing and measuring performance improvements are crucial steps in the code optimization process. By utilizing appropriate tools and methodologies, and interpreting metrics correctly, you can evaluate the effectiveness of your optimizations and achieve better performance for your software.
Best Practices for Continuous Code Optimization
Optimizing your code for better performance is not a one-time task. It requires continuous effort and dedication to maintain peak efficiency. Here are some best practices for incorporating code optimization into your development workflow:
Regular Code Reviews
Regular code reviews help identify potential performance bottlenecks and areas for improvement. They also encourage collaboration and knowledge sharing among team members. Schedule regular code reviews to ensure code optimization is consistently practiced.
Regular Refactoring
Refactoring involves restructuring code to make it more readable, maintainable, and efficient. Regular refactoring helps prevent performance degradation over time and improves scalability. Make it a practice to regularly refactor your codebase to ensure optimal performance.
Stay Up-to-Date with Industry Advancements
The technology landscape is constantly evolving, and staying up-to-date with industry advancements helps you identify new techniques and tools for code optimization. Attend conferences, webinars, and workshops to stay informed and updated on the latest developments.
Document Your Code
Properly documenting your code makes it easy to understand, maintain, and optimize. It also helps other team members to understand the code and collaborate more effectively. Use clear, concise, and accurate comments to document your codebase.
Use Version Control
Version control helps you track changes to your codebase, collaborate with other team members, and revert back to previous versions. It also makes it easy to identify changes that affect performance and optimize accordingly. Use a version control system to manage and track changes to your codebase.
Collaborate with Your Team
Collaboration is key to optimizing code for better performance. Encourage a team culture that prioritizes code optimization and promotes knowledge sharing. Share your code optimization techniques and learn from others in your team.
By implementing these best practices, you can maintain continuous code optimization and ensure long-term performance improvements.
Conclusion: Optimizing Your Code for Better Performance
In this article, we introduced the concept of code optimization and explored various strategies and techniques that can be used to optimize your code and improve efficiency. We discussed the benefits of optimizing your code, such as improved execution speed, reduced memory usage, and enhanced scalability.
To optimize your code, you first need to identify performance bottlenecks and determine areas that can be optimized for better efficiency. Techniques and tools such as profiling, benchmarking, and code review can help with this process.
Once you have identified areas for improvement, you can implement practical code optimization techniques such as algorithm optimizations, data structure optimizations, caching strategies, minimizing I/O operations, and reducing code redundancy.
Testing and measuring the effectiveness of code optimization techniques is crucial to ensuring long-term performance improvements. Load testing, stress testing, and profiling are some of the tools and methodologies that can be used for performance measurement.
Finally, we outlined best practices for maintaining continuous code optimization to ensure long-term performance improvements. Regular code reviews, refactoring, and staying up-to-date with industry advancements all play a role in optimizing code for better performance.
By applying the tweaks and tricks you have learned in this article, you can enhance your coding skills and productivity, ultimately achieving peak efficiency. Remember, continuous code optimization is key to achieving long-term success.
FAQ
Q: What is code optimization?
A: Code optimization refers to the process of improving the efficiency and performance of your code by making tweaks and implementing various techniques to achieve better execution speed, reduced memory usage, and enhanced scalability.
Q: Why is code optimization important?
A: Code optimization is crucial for achieving better performance and maximizing efficiency. By optimizing your code, you can improve execution speed, reduce memory usage, and enhance scalability, resulting in a more efficient and effective solution.
Q: How can I identify performance bottlenecks in my code?
A: You can identify performance bottlenecks in your code by analyzing and diagnosing performance issues using techniques such as profiling, benchmarking, and code review. These methods can help you pinpoint areas that can be optimized for better efficiency.
Q: What are some code optimization techniques I can implement?
A: Some code optimization techniques include algorithm optimizations, data structure optimizations, caching strategies, minimizing I/O operations, and reducing code redundancy. By implementing these techniques, you can optimize your code and achieve better performance.
Q: How do I test and measure performance improvements?
A: You can test and measure performance improvements by using tools and methodologies such as load testing, stress testing, and profiling. These methods can help you analyze the effectiveness of your optimizations and interpret performance metrics.
Q: What are some best practices for continuous code optimization?
A: Some best practices for continuous code optimization include regularly conducting code reviews, performing refactoring, staying up-to-date with industry advancements, maintaining documentation, utilizing version control, and fostering collaboration. These practices can help ensure long-term performance improvements.